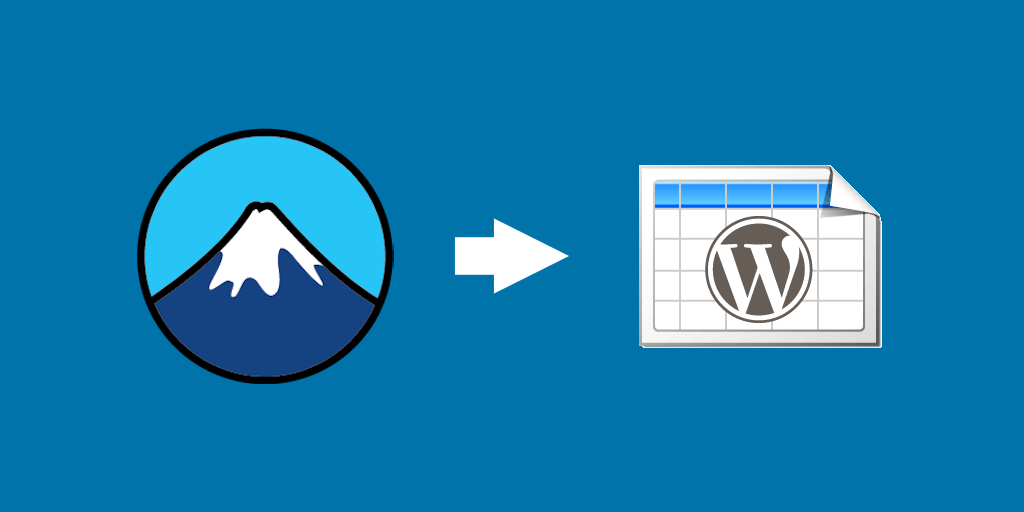
Here is a quick snippet of a PHP to store Contact Form 7 form submissions in TablePress tables. Make sure you update the form-to-table-ID mapping in $form_to_table_map
array to match your setup:
add_action(
'wpcf7_before_send_mail',
function ( $contact_form ) {
// Map Contact Form ID to Table ID.
$form_to_table_map = [
4 => 1,
];
$form_id = $contact_form->id();
if ( isset( $form_to_table_map[ $form_id ] ) && class_exists( 'TablePress' ) ) {
$table_id = $form_to_table_map[ $form_id ];
$table_model = TablePress::load_model( 'table' );
$table = $table_model->load( $table_id );
if ( is_array( $table['data'] ) ) {
$form_data = WPCF7_Submission::get_instance()->get_posted_data();
$table['data'][] = array_map( 'strval', $form_data );
$table_model->save( $table );
}
}
}
);
On each form submission it checks if the contact form ID matches an existing table ID and writes all field values to a new row in that table.
Be sure to register enough columns to fit all of contact form fields!
Hello,
I added your script but after submitting data from CF7:
1. no data was sent to tablepress (*).
2. it adds a code at the end of the url page such as #wpcf7-f11240-o2. So if you refresh the page, a resend message popups.
TablePress id = 2
so i updated script
That looks correct! Can you please confirm that your Contact Form ID is 4? If not, then change the
4
on the left from2
to the correct form ID or add another pair of form ID mapping to a TablePress ID.TablePress got data from CF7 but the table doesn’t display data in frontend because your code is adding 2 extra columns at the end of the table, one being empty “”
Example
Column 1: Name – Peter
Column 2: Email – email@email.com
Extra column: 1 – 11241
Extra column: “” – No
I had to go to the backend to refresh first, then unhide the related row in tablepress in order to display data frontend. strange behavior :)
This snippet works perfectly! Thank you a lot.
@Kaspars Thank you! This code works excellently!
Text-based fields work as expected, however I have a problem with arrays (checkboxes, radios, etc). I checked the database entry and this code just adds the text “Array” as the value. I have tried to fix it myself with some loops but with no success.
Can you provide an update that will parse the arrays into plain text?
See this post for how to parse the form fields. Looks like you could check if the data is an array and transform it into a string like this:
I haven’t tested this so I’m not sure if it works and what is the format that TablePress expects `$table[‘data’]` to be.
Thanks again Kaspars!
In my case I’ve implemented your suggestion like so:
For now i’m grabbing the specific fields that I know need to be imploded, some dropdowns and a group of checkboxes. I’m wondering, if I’m not using the
', '
separator can I completely remove the first argument? My temp solution is to make it an empty''
.Where does this snippet of code get added?
You could add it either to a new plugin that houses all the custom functionality for your site or using a plugin like Code Snippets to do that.